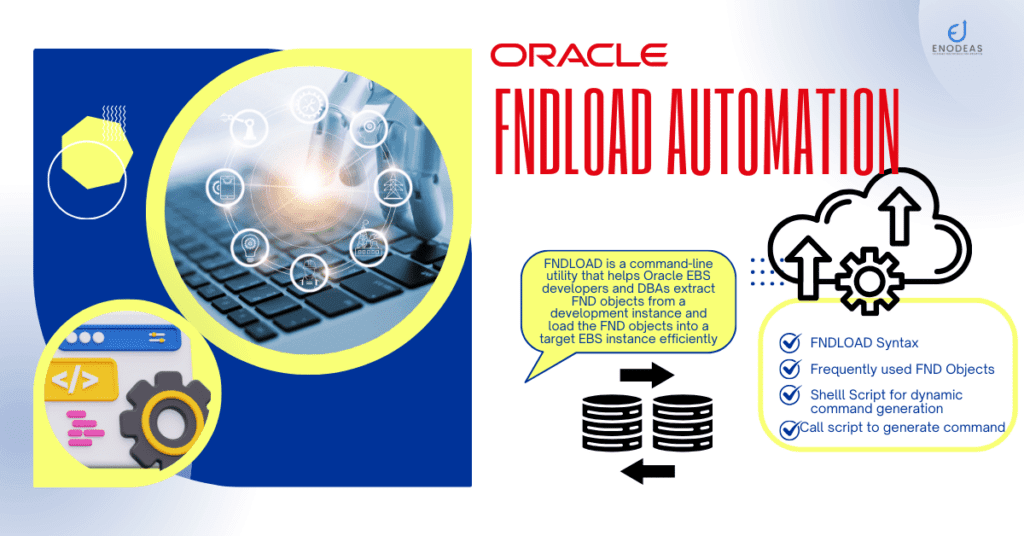
FNDLOAD in Oracle Apps: Automation Approach
What is FNDLOAD?
FNDLOAD is a command-line utility that helps Oracle EBS developers and DBAs extract FND objects from a development instance and load the FND objects into a target Oracle EBS instance efficiently. FND LOAD utility helps us to migrate FND objects like users, responsibility, menu, functions, concurrent programs, request sets, form personalizations, lookups etc. from source Oracle EBS application into a target Oracle EBS application instance.
I described details of all FND LOAD commands in my another blog post.
As a Oracle E-Business Suite (EBS) frequently deal with migrating configuration data across different environments. This article will provide a detailed understanding of FNDLOAD, including its syntax, use cases, best practices and how you can automatic command generation using script.
Table of Contents
Commonly Used FND LOAD Objects
Best Practices for Using FND LOAD
Why to Use FNDLOAD?
- Eliminates manual setup errors
- Provides a faster and consistent way to migrate configurations
- Works in batch mode, allowing automation
- Reduces dependency on database-level access for configuration migration
FND LOAD Command Syntax
FNDLOAD apps/apps_password 0 Y [MODE] [LCT_FILE][LDT_FILE][OPTIONS]
Parameter Description
Parameter | Description |
---|---|
apps/apps_password | Oracle Apps user credentials |
0 | Database connection mode (0 means Apps schema) |
Y | Log mode (Y = log messages to screen) |
<MODE> | DOWNLOAD (extracts data) or UPLOAD (migrates data) |
<LCT_FILE> | Loader control file (.lct) that defines object structure |
<LDT_FILE> | Data file (.ldt) containing extracted setup data |
[OPTIONS] | Optional parameters like – Warning=YES for logs |
Commonly Used FNDLOAD Objects
Object Type | LCT File | Example LDT File |
---|---|---|
Responsibilities | $FND_TOP/patch/115/import/afscursp.lct | xx_resp_key.ldt |
Users | $FND_TOP/patch/115/import/afscuur.lct | xx_resp_key.ldt |
Menus | $FND_TOP/patch/115/import/afsload.lct | menus.ldt |
Profile Options | $FND_TOP/patch/115/import/afspref.lct | profiles.ldt |
Concurrent Programs | $FND_TOP/patch/115/import/afcpreqg.lct | conc.ldt |
Best Practices for Using FND LOAD
- Always backup the destination instance impacted objects before uploading data
- Use version control (store LDT files in Git or SVN)
- Verify extracted data by opening the .ldt file in a text editor
- Run FND LOAD in test instance first before applying to production
- Always use shell scripts for bulk migration
Automating FNDLOAD with Shell Script
Let’s prepare a shell script to automate the FNDLOAD commands generation. You need to run the script with object type (user, responsibility, menu etc) and it will prepare the FNDLOAD command for that FND object type.
#!/bin/bash
# Oracle EBS Credentials
APPS_USER="apps"
APPS_PWD="p_apps_password"
# Define LCT files and OPTIONS for different object types
declare -A LCT_FILES
declare -A OPTIONS
LCT_FILES=(
["FND_RESPONSIBILITY"]="$FND_TOP/patch/115/import/afscursp.lct"
["FND_USER"]="$FND_TOP/patch/115/import/afscuur.lct"
["FND_MENU"]="$FND_TOP/patch/115/import/afsload.lct"
["FND_PROFILE"]="$FND_TOP/patch/115/import/afspref.lct"
["FND_CONCURRENT_PROGRAM"]="$FND_TOP/patch/115/import/afcpprog.lct"
["FND_REQUEST_GROUP"]="$FND_TOP/patch/115/import/afcpreqg.lct"
["FND_LOOKUP_TYPE"]="$FND_TOP/patch/115/import/aflvmlu.lct"
["FND_FORM"]="$FND_TOP/patch/115/import/afsload.lct"
["FND_FUNCTION"]="$FND_TOP/patch/115/import/afsload.lct"
["FND_MESSAGE"]="$FND_TOP/patch/115/import/afmdmsg.lct"
["ALR_ALERT"]="$ALR_TOP/patch/115/import/alr.lct"
["XDO_DS_DEFINITION"]="$XDO_TOP/patch/115/import/xdotmpl.lct"
)
OPTIONS=(
["FND_RESPONSIBILITY"]="RESP_KEY='YOUR_RESPONSIBILITY_KEY'"
["FND_USER"]="USER_NAME='YOUR_USER_NAME'"
["FND_MENU"]="MENU_NAME='YOUR_MENU_NAME'"
["FND_PROFILE"]="PROFILE_OPTION_NAME='YOUR_PROFILE_OPTION'"
["FND_CONCURRENT_PROGRAM"]="PROGRAM APPLICATION_SHORT_NAME='YOUR_APP_SHORT_NAME' CONCURRENT_PROGRAM_NAME='YOUR_PROGRAM_NAME'"
["FND_REQUEST_GROUP"]="REQUEST_GROUP REQUEST_GROUP_NAME='YOUR_REQUEST_GROUP_NAME' APPLICATION_SHORT_NAME='YOUR_APP_SHORT_NAME'"
["FND_LOOKUP_TYPE"]="LOOKUP_TYPE='YOUR_LOOKUP_TYPE' APPLICATION_SHORT_NAME='YOUR_APP_SHORT_NAME'"
["FND_FORM"]="FORM APPLICATION_SHORT_NAME='YOUR_APP_SHORT_NAME' FORM_NAME='YOUR_FORM_NAME'"
["FND_FUNCTION"]="FUNCTION FUNC_APP_SHORT_NAME='YOUR_APP_SHORT_NAME' FUNCTION_NAME='YOUR_FUNCTION_NAME'"
["FND_MESSAGE"]="FND_NEW_MESSAGES APPLICATION_SHORT_NAME='YOUR_APP_SHORT_NAME' MESSAGE_NAME='YOUR_MESSAGE_NAME'"
["ALR_ALERT"]="ALR_ALERTS APPLICATION_SHORT_NAME='YOUR_APP_SHORT_NAME' ALERT_NAME='YOUR_ALERT_NAME'"
["XDO_DS_DEFINITION"]="XDO_DS_DEFINITIONS APPLICATION_SHORT_NAME='YOUR_APP_SHORT_NAME' DATA_SOURCE_CODE='YOUR_DATA_SOURCE_CODE'"
)
# Object type alias mapping (for flexible input)
declare -A OBJECT_ALIASES=(
["RESPONSIBILITY"]="FND_RESPONSIBILITY"
["USER"]="FND_USER"
["MENU"]="FND_MENU"
["PROFILE"]="FND_PROFILE"
["CONCURRENT_PROGRAM"]="FND_CONCURRENT_PROGRAM"
["REQUEST_GROUP"]="FND_REQUEST_GROUP"
["LOOKUP_TYPE"]="FND_LOOKUP_TYPE"
["FORM"]="FND_FORM"
["FUNCTION"]="FND_FUNCTION"
["MESSAGE"]="FND_MESSAGE"
["ALERT"]="ALR_ALERT"
["DATA_SOURCE"]="XDO_DS_DEFINITION"
)
# Function to execute FNDLOAD
execute_fndload() {
local mode=$(echo "$1" | tr '[:lower:]' '[:upper:]') # Convert mode to uppercase
local object_type=$(echo "$2" | tr '[:lower:]' '[:upper:]') # Convert input to uppercase
local ldt_file="$3"
# Match object alias if necessary
if [[ -n "${OBJECT_ALIASES[$object_type]}" ]]; then
object_type="${OBJECT_ALIASES[$object_type]}"
fi
# Validate object type
if [[ -z "${LCT_FILES[$object_type]}" ]]; then
echo "Error: Unsupported object type '$2'!"
echo "Supported types: ${!LCT_FILES[@]}"
exit 1
fi
# Get the corresponding LCT file and OPTIONS
local lct_file="${LCT_FILES[$object_type]}"
local options="${OPTIONS[$object_type]}"
# Construct the FNDLOAD command
if [[ "$mode" == "DOWNLOAD" ]]; then
fndload_cmd="FNDLOAD $APPS_USER/$APPS_PWD 0 Y DOWNLOAD $lct_file $ldt_file $options"
elif [[ "$mode" == "UPLOAD" ]]; then
fndload_cmd="FNDLOAD $APPS_USER/$APPS_PWD 0 Y UPLOAD $lct_file $ldt_file"
else
echo "Error: Invalid mode! Use 'DOWNLOAD' or 'UPLOAD'."
exit 1
fi
# Display full command before execution
echo "Generated FNDLOAD Command:"
echo "$fndload_cmd"
# Execute the command
#eval $fndload_cmd
}
# Main script execution
if [[ $# -lt 3 ]]; then
echo "Usage: ./fndload_commands.sh <DOWNLOAD|UPLOAD> <OBJECT_TYPE> <LDT_FILE>"
echo "Example: ./fndload_commands.sh DOWNLOAD menu menu.ldt"
exit 1
fi
execute_fndload "$1" "$2" "$3"
FNDLOAD Automation[appxxtst@xxebs05]$ fndload_commands.sh DOWNLOAD responsibility xx_resp_key.ldt
Generated FNDLOAD Command:
FNDLOAD apps/p_apps_password 0 Y DOWNLOAD $FND_TOP/patch/115/import/afscursp.lct xx_resp_key.ldt RESP_KEY='YOUR_RESPONSIBILITY_KEY'
[appxxtst@xxebs05]$ fndload_commands.sh UPLOAD responsibility xx_resp_key.ldt
Generated FNDLOAD Command:
FNDLOAD apps/p_apps_password 0 Y UPLOAD $FND_TOP/patch/115/import/afscursp.lct xx_resp_key.ldt
fndload_commands.sh to generate commandYou can generate any FND LOAD command using the above shell script. Please let me know if you want Python script for this.
Conclusion
FND LOAD is an essential tool for Oracle EBS administrators and developers, enabling efficient and error-free migrations of configuration data. By leveraging its powerful capabilities, you can automate and streamline setup processes across multiple instances.
Pingback: Complete Guide to FNDLOAD Commands in Oracle Apps R12