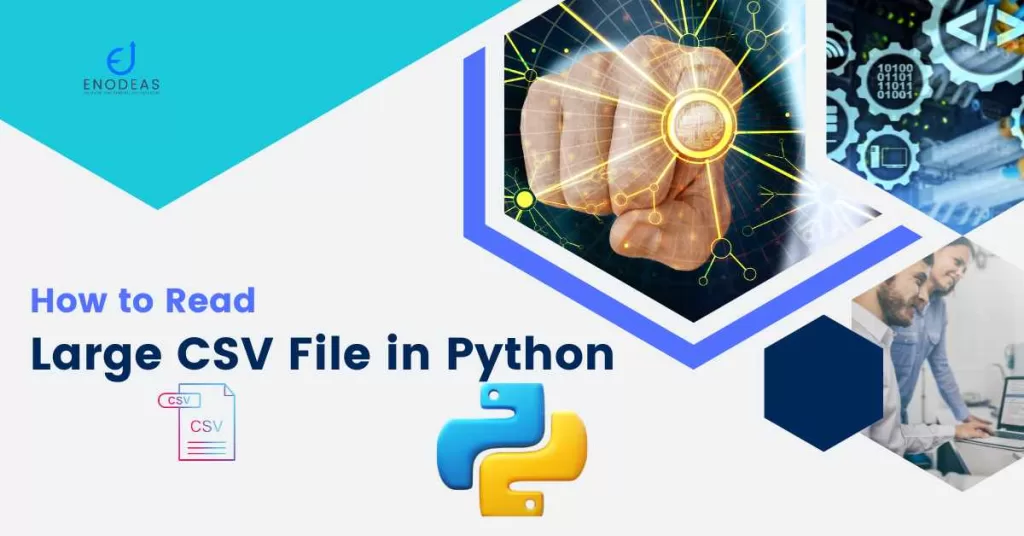
Read Large CSV File in Python: Effective ways
Handling very large CSV files efficiently is a formidable challenge in the world of data processing. In this blog post, we will explore five top-notch approaches to read large CSV file in Python to tackle this task head-on. By the end of this comprehensive guide, you’ll have a clear understanding of each approach’s advantages and disadvantages, enabling you to make informed choices for your data processing needs.
Here are the five best way to Read Large CSV File in Python:
Following are the best way to read large csv file in python.
- Pandas: Pandas is a popular Python library for data analysis and manipulation. It offers a convenient and efficient way to read and process CSV files, even large ones. However, Pandas can be memory-intensive, so it may not be the best option for very large files.
- Dask: Dask is a distributed computing library that can be used to scale Pandas workloads to multiple machines. This makes it a good option for reading and processing very large CSV files. However, Dask can be more complex to use than Pandas.
- Modin: Modin is a distributed computing library that can be used to accelerate Pandas workloads. It is similar to Dask, but it is designed to work with Pandas dataframes. This makes it a good option for users who are already familiar with Pandas.
- Vaex: Vaex uses memory-mapped DataFrames to efficiently process datasets that are larger than the available RAM. Memory-mapped DataFrames map the file to memory, which allows Vaex to access the data quickly and efficiently.
- PySpark: PySpark is a distributed computing framework. PySpark can be used to process large datasets that are too large to fit into a single machine’s memory. Python read large csv file fast using PySpark.
1. Pandas – Best Way to Read Large CSV File in Python
Pandas is a popular Python library for data analysis and manipulation. It is versatile and easy to use, and it offers a convenient way to read and process CSV files, even large ones. However, Pandas can be memory-intensive, so it may not be the best option for very large files.
Here is an example of how to load a large CSV file using Pandas:
import pandas as pd
df = pd.read_csv('very_large_file.csv')
Read CSV FileAdvantages of using Pandas:
- Simplicity: Pandas provides a user-friendly interface, making it easy to learn and use.
- Data manipulation: Pandas is ideal for data cleaning and transformation tasks, such as filtering, sorting, and aggregating data.
Disadvantages of using Pandas:
- Memory intensive: Pandas can be memory-intensive, especially when working with large files.
- Slower processing: Pandas may be slower than other libraries when processing very large files.
Pandas, a beloved data manipulation library, employs chunking for large files and it resolves memory issues.
Example:
import pandas as pd
for chunk in pd.read_csv('large_file.csv', chunksize=1000):
PythonPandas is a powerful and versatile tool for data analysis and manipulation. It is a good choice for users of all skill levels, and it is widely supported by other Python libraries and tools.
2. Dask – Scaling Pandas for Handling Large CSV Datasets
Dask is a Python library for parallel computing. It extends the capabilities of Pandas to handle large datasets that are too large to fit into memory. Dask excels in distributing computations across cores to improve performance.
Here is an example of how to read a large CSV file using Dask:
import dask.dataframe as dd
df = dd.read_csv('large_dataset.csv')
PythonAdvantages of using Dask:
- Seamless scalability: Dask can scale seamlessly to handle large datasets.
- Improved performance: Dask utilizes parallel processing to improve the performance of data processing tasks.
Disadvantages of using Dask:
- Steeper learning curve: Dask can have a steeper learning curve than Pandas, especially for users who are new to parallel computing.
Safety guideline:
When using Dask to read large CSV files, it is important to be aware of the memory requirements. Dask may need to store the entire file in memory in order to process it. If the file is too large to fit into memory, you may need to use a different approach, such as reading the file in chunks or using a distributed computing platform like Apache Spark.
Overall, Dask is a powerful tool for scaling Pandas to handle large datasets. It is a good choice for users who need to process large datasets and improve the performance of their data processing workflows.
3. Modin – Best Way to Read Large CSV File in Python
Modin is a Python library that can seamlessly switch between Pandas and Dask under the hood, depending on the size of the dataset. This makes it a good choice for both small and large datasets.
Here is an example of how to read a large CSV file using Modin:
import modin.pandas as mpd
df = mpd.read_csv('large_dataset.csv')
PythonAdvantages of using Modin:
- Seamlessly transitions between Pandas and Dask for optimal performance.
- Minimal code changes required for implementation.
Disadvantages of using Modin:
- Modin may not support all Pandas functions.
Safety guideline:
When using Modin to read large CSV files, it is important to be aware of the memory requirements. Modin may need to store a portion of the file in memory in order to process it. If the file is too large to fit into memory, you may need to use a different approach, such as reading the file in chunks or using a distributed computing platform like Apache Spark.
4. Vaex – Best Way to Read Large CSV File in Python
Vaex is a Python library that uses memory-mapped DataFrames to efficiently process datasets that are larger than the available RAM. Memory-mapped DataFrames map the file to memory, which allows Vaex to access the data quickly and efficiently.
Here is an example of how to read a large CSV file using Vaex:
import vaex
df = vaex.from_csv('large_dataset.csv', convert=True)
PythonAdvantages of using Vaex:
- Extraordinary performance on datasets exceeding available memory.
- Memory-mapping leads to minimal memory consumption.
Disadvantages of using Vaex:
- Limited support for data manipulation operations compared to Pandas.
5. PySpark – Best Way to Read Large CSV File in Python
Apache PySpark is a Python library for Apache Spark, a distributed computing framework. PySpark can be used to process large datasets that are too large to fit into a single machine’s memory. You can easily read csv file with more than 10 gigabytes easily using PySpark.
PySpark needs to install in your local machaine after doing some extra steps:
- Install PySpark using pip or Conda.
- Set the
SPARK_HOME
andPYSPARK_PYTHON
environment variables. - Verify the installation by running a simple PySpark program.
Please refer to my earlier blog post about PySpark Installation Process.
Here is an example of how to read a large CSV file using PySpark:
from pyspark.sql import SparkSession
csv_file_name = "large_csv_file.csv"
spark = SparkSession.builder.appName("large_file_read").getOrCreate()
df = spark.read.csv(csv_file_name, header=True)
PythonAdvantages of using PySpark:
- Scales effortlessly to handle massive datasets.
- Leverages distributed computing for unparalleled performance.
Disadvantages of using PySpark:
- Overheads associated with setting up and managing a Spark cluster.
Conclusion: Best Way to Read Large CSV File in Python
The best approach of how to read large CSV file will depend on the specific needs of your task. For moderately sized datasets, Python libraries like Pandas and Dask are good choices. When facing mammoth files, Vaex and PySpark step into the limelight. Modin, on the other hand, seamlessly adapts between Pandas and Dask, providing a versatile solution.
Here are some additional safety guidelines to keep in mind for best way to read large csv file in python:
- Be aware of the memory requirements. When using a Python library to read a large CSV file, it is important to be aware of the memory requirements. If the file is too large to fit into memory, you may need to use a different approach, such as reading the file in chunks or using a distributed computing platform.
- Handle errors gracefully. When reading large CSV files, it is important to handle errors gracefully. For example, if the file is corrupted or contains invalid data, you should handle these errors without crashing your program.
- Use a timeout mechanism. When reading large CSV files, it is important to use a timeout mechanism. This will prevent your program from hanging indefinitely if the file is taking too long to read.
Pingback: What is Parquet File Format – Enodeas
Pingback: 10 Minutes to Pandas [Python Tutorial]: A Complete Guide – Enodeas
Pingback: How to Install PySpark on Your Windows Machine Effortlessly – Enodeas