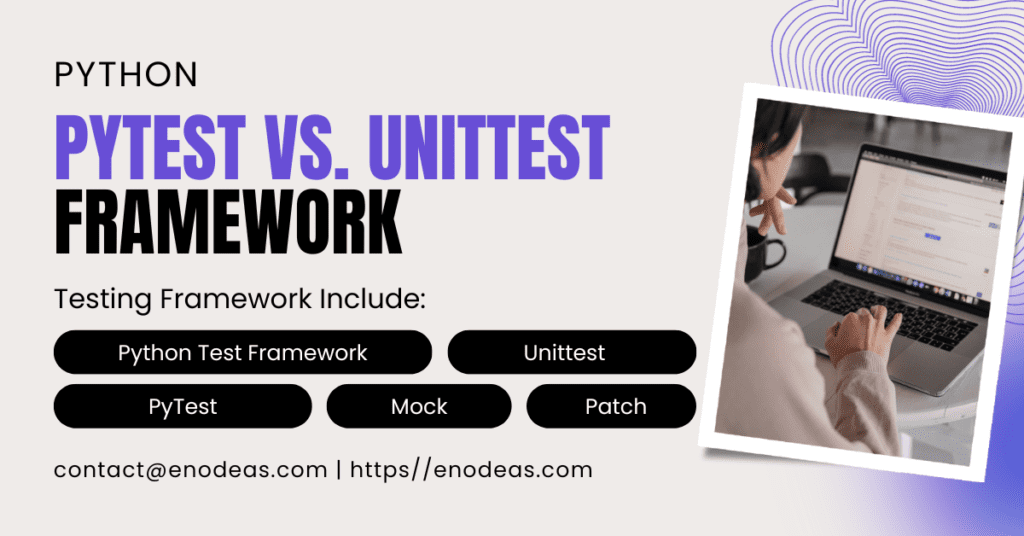
Complete Guide to Learn PyTest and Unittest in Python
You always want to write robust code, which is key, and that’s where Python Unit testing frameworks (PyTest vs Unittest) come in. There is always an issue when you have multiple options: which do you choose, PyTest or Unittest? As both testing frameworks bring different strengths to the table, you need to know the functionalities, benefits, and weaknesses of both PyTest and Unittest. Let’s dive into the functionalities of PyTest vs unit test. We’ll examine how to conduct tests using each framework and then compare them. You can review our other articles on our blog post page.
PyTest Vs Unittest in Simple Words
Let’s take Python testing for a spin! Imagine two friends, PyTest and Unittest, both helping you build robust code. You will see the difference of pytest vs unit test.
PyTest: Think “easy breezy.” No fancy setup, just name your tests with “test_” and go! It’s flexible, loves plugins for extra tricks, and keeps things clear.
Unittest: Your built-in buddy, always there. It likes structure, with test classes and all, but keeps things simple. No need for extra tools, just write your tests and let it run.
So, who to choose Unittest vs Pytest? For big projects, PyTest’s flexibility shines. For smaller ones, Unittest’s simplicity rocks. No matter who you pick, both friends make sure your code stands tall!
What is Python PyTest?
Pytest is a powerful testing framework for Python, streamlining the testing process. It stands out for simplicity and scalability, enabling efficient unit testing and functional testing. With a user-friendly syntax, Pytest simplifies writing and executing tests. Its fixture system aids in setting up preconditions, enhancing test modularity. The framework’s detailed reporting and rich ecosystem contribute to effective test management.
PyTest fosters code readability and encourages the use of Pythonic idioms, promoting clean and concise test scripts. This framework is essential for ensuring code reliability, making it a go-to choice in the Python programming landscape.
What is Python Unittest?
Unittest, a built-in Python tool, is your ally for creating focused unit tests. These tests act like inspecting each brick in a wall to ensure overall strength.
Imagine crafting a Python Unittest as weaving a detective story:
- Setup: Collect clues (data, objects) needed by the code.
- Exercise: Run the code like a magnifying glass, scrutinizing its output.
- Assert: Compare results to expectations, akin to checking if the culprit matches the description.
Failed tests pinpoint where to investigate, simplifying debugging. Unittests:
- Catch bugs early: Nipping issues in the bud before they escalate.
- Enhance code quality: Confirming individual parts function as intended.
- Refactor confidently: Assuring changes won’t break anything.
Though Python Unittest lacks flashy features, it’s a dependable toolbox, always ready to assist in constructing robust Python code.
How to Use Python PyTest to Test Code?
In order to use PyTest in Python You need to install PyTest using pip installer.
pip install pytest
pip install pyestLet’s consider a simple Python function that calculates the square of a number. We’ll create a test for this function using pytest.
#square.py
def calculate_square(number):
return number ** 2
Example Test Script for PyTestIn the above example, we define a function calculate_square for calculating square of a number.
Let’s see how we can use PyTest to test the basic functionality of the above function.
#test_square.py
from square import calculate_square
def test_calculate_square():
# Test case 1: Check the square of a positive number
result = calculate_square(9)
assert result == 81
# Test case 2: Check the square of zero
result = calculate_square(0)
assert result == 0
# Test case 3: Check the square of a negative number
result = calculate_square(-5)
assert result == 25
Pytest vs unittestIn the above example, we demonstrates a basic use of pytest for testing a function that calculates the square of a number. using the assert statement to check if the function produces the expected results. If function returns expected value PyTest to mark the test case PASS otherwise it will fail the test case.
Simply execute the below secript to run the pytest
PS C:\Python\Square> pytest -v -s
============== test session starts ====================================
platform win32 -- Python 3.9.13, pytest-7.1.2, pluggy-1.3.0 -- C:\Users\enodeas\anaconda3\python.exe
cachedir: .pytest_cache
metadata: {'Python': '3.9.13', 'Platform': 'Windows-10-10.0.22621-SP0', 'Packages': {'pytest': '7.1.2', 'pluggy': '1.3.0'}, 'Plugins': {'anyio': '3.7.1', 'html': '4.0.2', 'metadata': '3.0.0', 'redis': '3.0.2', 'xdist': '3.3.1'}, 'JAVA_HOME': 'C:\\Program Files\\Java\\jdk1.8.0_351'}
rootdir: C:\Python\Square
plugins: anyio-3.7.1, html-4.0.2, metadata-3.0.0, redis-3.0.2, xdist-3.3.1
collected 1 item
test_square.py::test_calculate_square PASSED
========================== 1 passed in 0.01s ==========================
PS C:\Python\Square>
Execution of PyTestPytest discovers all the test files, reporting the test results with detailed information. By default PyTest identify test files, classes and test function as per the below naming convention.
python_files = test_*
python_classes = *Tests
python_functions = test_*
PyTest Config fileIf you want different way of identify the test script and test function you have to create a pytest.ini file in test home directory. The pytest.ini file is a powerful tool that is used to customize the way that PyTest runs your tests. In the below config file PyTest will identify python files, function and class name start with Check.
python_files = check_*
python_classes = Check*
python_functions = check_*
PyTest Config FileHow to Write Test Scripts with Unittest?
Writing tests with unitttest in Python involves creating test cases to check if your code works as expected. Start by importing unittest. Then, make a class that inherits from unittest.TestCase. Inside this class, write test methods. These methods should check different aspects of your code using simple assertions like self.assertEqual().
Here’s a step-by-step guide:
- Begin by importing the unittest module
- Create a Test Case Class
- Write Test Methods
- Use Assertion Methods
- Run Tests
- Execute Tests
For example, if you have a function calculate_square that squares a number, a test could ensure it works for positive, zero, and negative numbers. After writing your test methods, use unitttest.main() to run your tests. If everything is correct, you’ll see an OK message; otherwise, it’ll point out which tests failed. Writing tests helps catch bugs early, improve code quality, and give you confidence when making changes.
#1. Import Unittest library
import unittest
from square import calculate_square
#2. Create Test case
class TestSquare(unittest.TestCase):
# 3. Write test method
def test_calculate_square(self):
# Test case 1: Check the square of a positive number
result = calculate_square(9)
#4. assert method
self.assertEqual(result, 81)
# Test case 2: Check the square of zero
result = calculate_square(0)
self.assertEqual(result, 0)
# Test case 3: Check the square of a negative number
result = calculate_square(-5)
self.assertEqual(result, 25)
#Run Test
if __name__ == '__main__':
unittest.main()
unittest vs pytest In this example, we created a class TestSquare that inherits from unittest.TestCase. That class is to contain your test methods. The assertions, such as self.assertEqual, are used to check if the actual output matches the expected output.
At the end, execute the following command to run the unittest tests in Python terminal:
PS C:\Python\Square> python test_squareut.py -v
test_calculate_square (__main__.TestSquare) ... ok
----------------------------------------------------------------------
Ran 1 test in 0.000s
OK
PS C:\Python\Square>
unittest vs pytestPython Pytest vs Unittest
Stuck between PyTest vs Unittest for your Python testing? Fear not, code warriors! Let’s break down their top 5 differences of unittest vs pytest so you can pick your perfect match:
- Style Symphony:
- Pytest: Embrace the carefree spirit – just prefix functions with “test_” for instant magic. No classes, no hassle, just testing brilliance.
- Unittest: Opt for a more formalized approach. Craft test classes and methods with a structured finesse. Picture it as a methodical detective, meticulously examining each clue. ️
- Adaptability Arena:
- Pytest: A true chameleon, molding to your desires. Need intricate fixtures or personalized reports? Enter its plugin playground for limitless customization.
- Unittest: A steadfast companion, sticking to built-in features for simplicity and familiarity. Think of it as a reliable toolbox, always equipped with the essentials.
- Learning Landscape:
- Pytest: A steeper initial climb, especially with extensive customization options. Once mastered, you’ll wield testing prowess like a ninja!
- Unittest: A gentle slope for beginners. Its structured nature facilitates quick comprehension, making it perfect for an initial testing venture. ♀️
- Project Prowess:
- Pytest: Unleashes superhero scaling capabilities, excelling in large, intricate projects with diverse testing demands.
- Unittest: Tailored for smaller endeavors or those valuing a clear, structured path. Imagine it as the dependable sidekick, always ready to support your testing expedition.
- Community Cadence:
- Pytest: Revels in a lively community, offering a plethora of plugins, tutorials, and support. Navigating the testing jungle is a collaborative adventure!
- Unittest: Intrinsic to the Python family, providing inherent support. External help is unnecessary, but resources abound for those seeking additional guidance.
Choose Python Unittest Vs PyTest
In the end, the ideal choice hinges on your project and preferences. Pytest, the adaptable virtuoso, excels in grand testing productions. Unittest, the steadfast ally, shines in smaller arenas and appeals to beginners. So both (Unittest vs PyTest) the frameworks are very useful of Python programmer like you. Remember, both frameworks are committed to fortifying your Python code with strength and confidence!
Benefits of PyTest vs Unittest
Pytest and Unittest offer distinct advantages in Python testing. Pytest’s minimalist syntax streamlines testing with a “name it, run it” approach, reducing complexity and enhancing readability. Its rich fixture and plugin ecosystem empower developers to tailor testing environments, fostering adaptability in large projects. Pytest’s dynamic nature and seamless test discovery further contribute to its appeal. On the other hand, Unittest, integrated into Python, provides a structured and familiar approach ideal for smaller projects. With a gentle learning curve, it’s approachable for beginners, offering stability and inherent support. Ultimately, the choice hinges on project needs, scale, and the team’s preference for flexibility or tradition.
In summary, Pytest excels in flexibility, adaptability, and a vibrant ecosystem, making it ideal for dynamic and evolving projects. On the other hand, Unittest offers a structured, integrated approach, making it suitable for smaller projects and those preferring a more traditional testing framework. The choice between them depends on project requirements, preferences, and the development team’s familiarity with each framework.